Centralized Configuration Management using Azure App Configuration: Implementing Custom Offline Cache
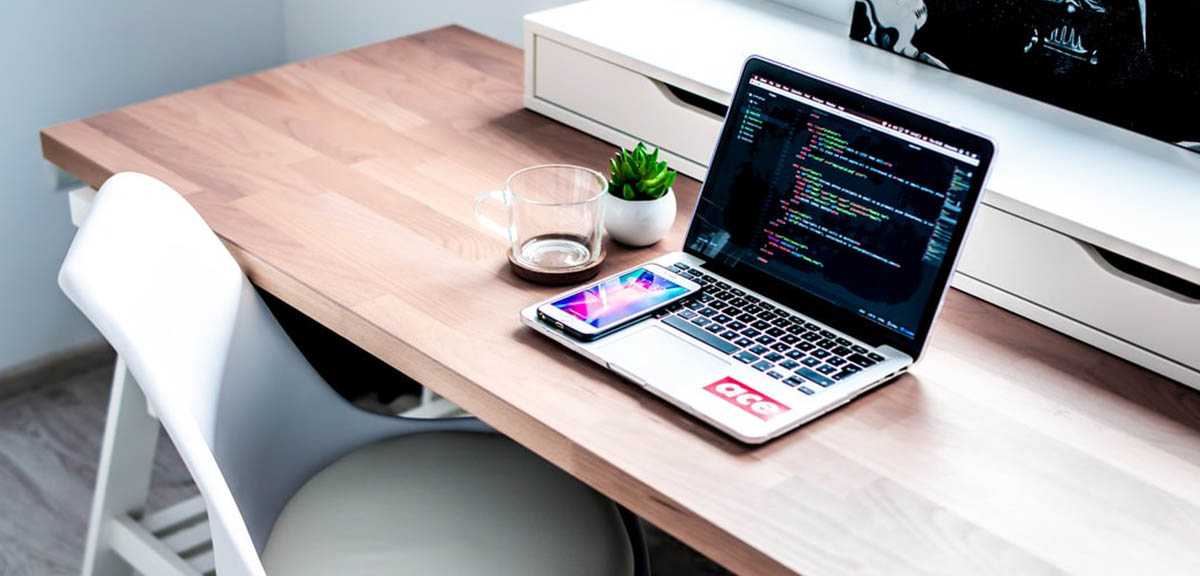
Centralized Configuration Management using Azure App Configuration Series
- Introduction
- Using Managed Identities to Access Azure App Configuration
- Setting Up Dynamic Refresh for Configuration Values
- Setting Up Offline Caching
- Implementing Custom Offline Cache (This Article)
- Using Azure Key Vault Side-by-Side
- Local Debugging When Using Managed Identities to Access Azure App Configuration
In the previous article we looked into how we can configure Offline Caching for Azure App Configuration. In this article we will look at how we can create a custom Offline Caching implementation. To do this we need to implement the IOfflineCache
interface. This interface has 2 methods we need to implement. Export()
method will cache the data and Import()
will retrieve the cached data.
Let’s create our own simple IOfflineCache
implementation that stores the cached app configuration in a json file in our local file system. Check the code segment below.
using Microsoft.Extensions.Configuration.AzureAppConfiguration;
using Microsoft.Extensions.Hosting;
using System.IO;
namespace MusicStore.Web.Cache
{
public class LocalFileOfflineCache : IOfflineCache
{
private readonly string _cacheFilePath;
public LocalFileOfflineCache(IHostEnvironment env)
{
_cacheFilePath = Path.Combine(env.ContentRootPath, "offline_cache.json");
}
public void Export(AzureAppConfigurationOptions options, string data)
{
File.WriteAllText(_cacheFilePath, data);
}
public string Import(AzureAppConfigurationOptions options)
{
return File.ReadAllText(_cacheFilePath);
}
}
}
In the LocalFileOfflineCache
implementation of IOfflineCache
interface what we are simply trying to do is to use a JSON file in the local disk as the offline cache. In the Export()
method we save it to the JSON file and in the Import()
method we retrieve the cached data from the JSON file.
How we configure this to be used is simple, We just need to supply the SetOfflineCache()
method with an instance of the LocalFileOfflineCache
instance and pass in the required IHostEnvironment
parameter.
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder => {
webBuilder.UseStartup<Startup>();
})
.ConfigureAppConfiguration((context, config) => {
var settings = config.Build();
var appConfigEndpoint = settings["AppSettings:AppConfiguration:Endpoint"];
if (!string.IsNullOrEmpty(appConfigEndpoint))
{
var endpoint = new Uri(appConfigEndpoint);
config.AddAzureAppConfiguration(options =>
{
...
options
// Use the custom IOfflineCache implementation
.SetOfflineCache(new LocalFileOfflineCache(context.HostingEnvironment));
});
}
});
As you can see if you require a custom cache implementation for your Azure App Configuration you can easily implement your own.
Summary
In this article we looked at how we can implement our own custom implementation of IOfflineCache
and use it for the Azure App Configuration Offline cache. You can find the source code in the Azure App Configuration Custom Offline Cache Example GitHub repository. In the next article we will look at how we can use Azure Key Vault and combine it with Azure App Configuration.
You Might Also Like
← Previous Post