Feature Flags for ASP.Net Core Applications: Advanced Uses of Azure App Configuration for Feature Management
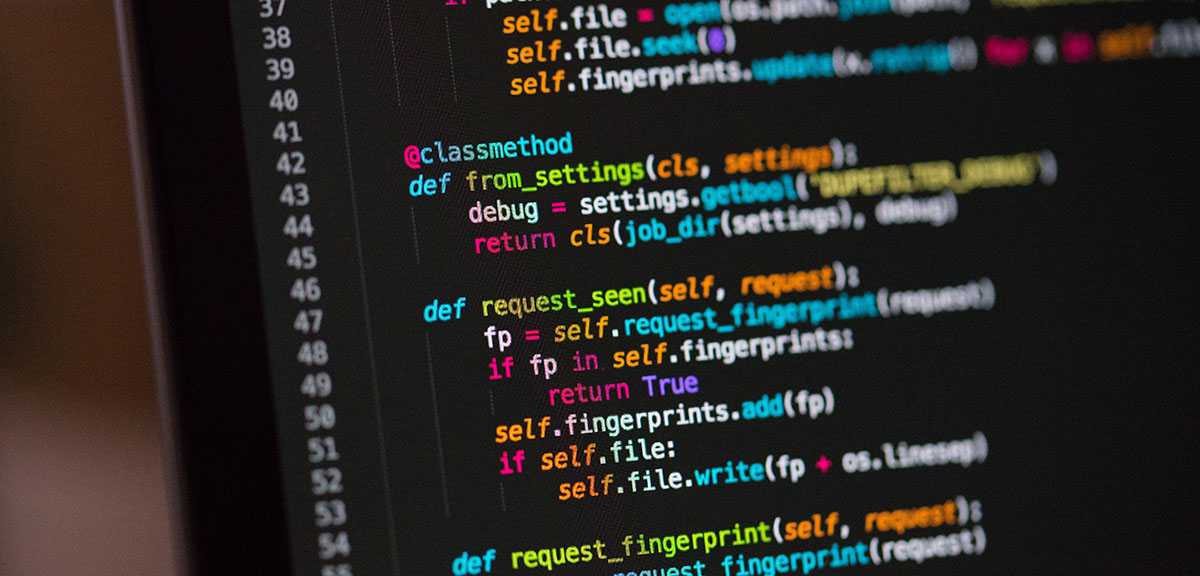
Feature Flags for ASP.Net Core Applications Series
- Introduction to using Microsoft.FeatureManagement Library
- Combining Multiple Feature Flags to Control Feature Exposure
- Using Complex Feature Flags with Feature Filters
- Implementing Custom Feature Filters
- Handling Action Disabled by Feature Flags
- Using Azure App Configuration for Feature Management
- Advanced Uses of Azure App Configuration for Feature Management (This Article)
In the last article on Using Azure App Configuration Feature Management in ASP.Net Core Applications, we talked about how we can manage feature flags using Azure App Configuration. In this article we will look at couple of important advanced capabilities of Azure App Configuration feature management.
Reducing Cache Expiration Time to Decrease the Refresh Time of Feature Flags
When you use Azure App Configuration with your application when you update the feature flag on the Azure App Configuration, it will take a few seconds to update the change in your application. This may take up to 30 seconds in some cases. This is because there is caching going on. The feature flags are cached once retrieved from App Configuration and once the cache is expired the feature flags are fetched from the cloud again and updated. By default, there is a 30 second cache expiry time set. We can change this value and make the update happen quickly.
Where we can do this is in the Program.cs
file inside ConfigureAppConfiguration()
method. See the code example below.
namespace MusicStore.Web
{
public class Program
{
...
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder => {
webBuilder.UseStartup<Startup>();
})
.ConfigureAppConfiguration((context, config) => {
var settings = config.Build();
var appConfigConnection = settings["ConnectionStrings:AppConfiguration"];
if (!string.IsNullOrEmpty(appConfigConnection))
{
config.AddAzureAppConfiguration(options =>
{
options
.Connect(settings["ConnectionStrings:AppConfiguration"])
.UseFeatureFlags(opt => {
opt.CacheExpirationTime = TimeSpan.FromSeconds(5); // Set cache expiry to 5 seconds
});
});
}
});
}
}
Here in this example, in AddAzureAppConfiguration()
method we use UseFeatureFlags()
method to enable feature management for Azure App Configuration. Here in the UseFeatureFlag()
method we can pass a FeatureFlagOptions
instance and configure few details. One such detail is CacheExpirationTime
which is a TimeSpan
object. Here in this example we set a TimeSpan
of 5 seconds as the
CacheExpirationTime
reducing the default expiration time.
Now if you run the application, then update a feature flag and try refreshing the application, you will notice that the feature flags are updated much faster than before.
Using Labels for Multi-Dimensional Feature Flags
Azure App Configuration can tag Configuration settings and feature flags with Labels. This label gives capabilities to have multiple values for the same feature flag. This opens several possibilities. For example,
- You have multiple environments for your application, like Dev, Test and Stage environments you can maintain Feature flags for all these environments in a single Azure App Configuration instance.
- You have an application that is distributed across multiple geographic regions (E.g. Music Store app is deployed to Asia, Europe and US regions) you can release features to deployed regions one by one progressively enabling up feature for certain features etc.
So, you can see, using labels for your feature flags is powerful. Let’s take the scenario where our Music Store application is distributed into multiple regions and we need to maintain feature flags for each region.
We can use a label we define on Azure App Configuration to filter the feature flags and select the feature flags that matches the label. This how we do it.
namespace MusicStore.Web
{
public class Program
{
...
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder => {
webBuilder.UseStartup<Startup>();
})
.ConfigureAppConfiguration((context, config) => {
var settings = config.Build();
var appConfigConnection = settings["ConnectionStrings:AppConfiguration"];
if (!string.IsNullOrEmpty(appConfigConnection))
{
config.AddAzureAppConfiguration(options =>
{
options
.Connect(settings["ConnectionStrings:AppConfiguration"])
.UseFeatureFlags(opt => {
// Use the environment variable set on Azure App Service as the label.
opt.Label = Environment.GetEnvironmentVariable("REGION_NAME")?.ToLowerInvariant().Trim().Replace(' ', '_');
});
});
}
});
}
}
Here we set the Label property on the FeatureFlagOptions
object in the UseFeatureFlag
method. What I am setting the value to is from the environment variable called REGION_NAME that is available in Azure App Services instances. For local development, I set the environment variable on my Visual Studio 2019. And then we need to go to the Azure Portal and create the Labels for Feature Flags.
Go to the Azure App Configuration instance we created and the on an already existing feature flag, click on the ellipsis button and from the context menu, click on Edit link.
Then on the edit feature flag blade add a new Label. In this example I am defining a simple feature flag with the label local
and clicked apply.
And in the scenario, I am planning to deploy the Music Store application in Southeast Asia and South Central US regions. So, I am creating new labels with different values for the feature flag.
So here in the Promotion feature flag, I have 3 labels now.
- local - Simple flag. Set to On. For local development
- south_central_us – Simple flag. Set to Off. For the instances deployed to South Central US
- southeast_asia – Conditional flag. Uses MusicStore.Browser feature filter and allowed for Chrome and IE browser. For the instances deployed to Southeast Asia.
So, you can see, you can use different types of feature filters, simple or conditional with different types of feature filters for different labels. This adds a huge amount of flexibility when you manage your feature flags. So run the application and you can change the value of the REGION_NAME environment variable on your Visual Studio and test the implementation.
Summary
In this article we looked at couple of advance uses of Azure App Configuration. Setting a custom cache expiry will help you change when you want to update the feature flags when they change. To make the change available much faster than default. And with Labels, it allows you to support many different deployment scenarios adding multiple dimensions for a single feature flag.
You Might Also Like
← Previous Post