Feature Flags for ASP.Net Core Applications: Combining Multiple Feature Flags to Control Feature Exposure
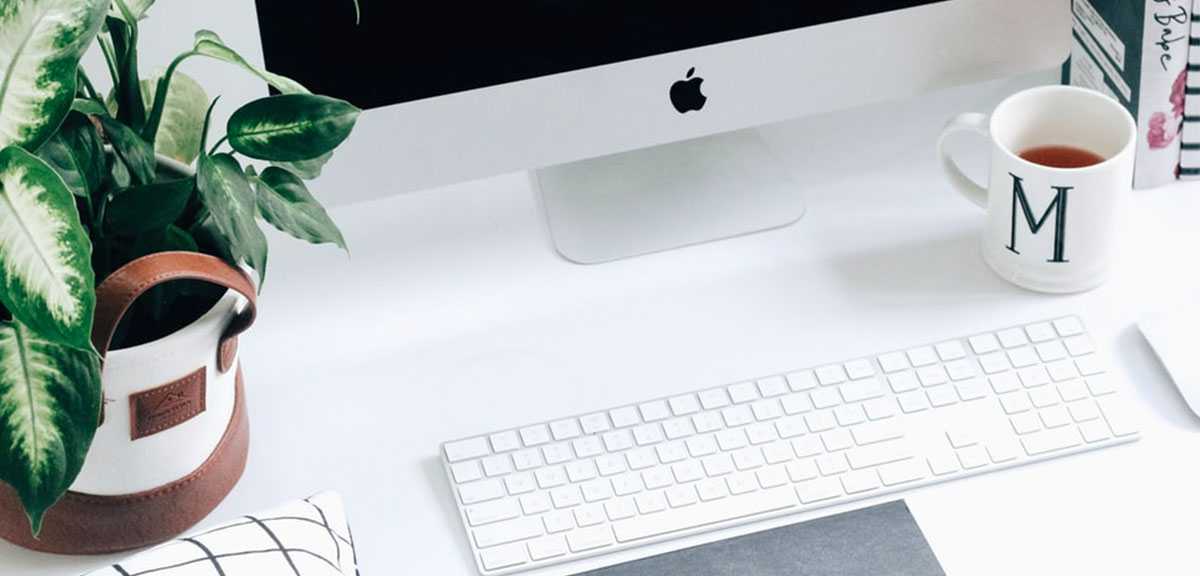
Feature Flags for ASP.Net Core Applications Series
- Introduction to using Microsoft.FeatureManagement Library
- Combining Multiple Feature Flags to Control Feature Exposure (This Article)
- Using Complex Feature Flags with Feature Filters
- Implementing Custom Feature Filters
- Handling Action Disabled by Feature Flags
- Using Azure App Configuration for Feature Management
- Advanced Uses of Azure App Configuration for Feature Management
In the previous article, we looked into how we can implement feature flags to control the exposure to features for ASP.Net Core MVC application using Microsoft.FeatureManagement
and Microsoft.FeatureManagement.AspNetCore
libraries. We used the Music Store example to demonstrate how we can use simple feature flags to control feature exposure.
In this article we will see how we can combine multiple feature flags to control feature exposure. We can do this for MVC controller action methods using FeatureGate
attribute and for Razor Views using the provided <feature>
tag helper.
In the Music Store example, we used in the previous article, to enable the Promotions view we only used the Promotion
feature flag. We then used Promotion.Discounts
flag to enable a 25% discount for all products shown in Promotions view. But what if we need to enable the Promotions page only when Promotion and Promotion.Discounts
feature flags are set to true? We can do this quite easily by combining multiple feature flags to enable a single feature.
Combining Multiple Feature Flags for Action Methods using FeatureGate Filter
In the previous example we only supplied the name of the feature flag to the FeatureGate filter attribute. But it has couple of more options.
FeatureGate
filter can accept multiple feature flag namesFeatuteGate
filter can also acceptRequirementType
enum as a parameter
RequirementType
has 2 enum values. All
and Any
namespace Microsoft.FeatureManagement
{
public enum RequirementType
{
Any = 0, // The enabled state will be attained if any feature in the set is enabled.
All = 1 // The enabled state will be attained if all features in the set are enabled.
}
}
If the RequirementType
is set to Any
if you have multiple feature flags if any one of them is set to true or evaluates to true, the feature flag will be enabled.
If the RequirementType
is set to All
, if you have multiple feature flags all of them needs to be set to true or needs to evaluate to true for the feature flag to be enabled.
In our example we want to make sure both Promotion
and Promotion.Discounts
flags are set to true to show the Promotions page. So, we will do something like this.
using Microsoft.FeatureManagement.Mvc;
namespace MusicStore.Web.Controllers
{
public class HomeController : Controller
{
...
[FeatureGate(RequirementType.All, Features.Promotions, Features.PromotionDiscounts)]
public async Task<IActionResult> Promotions()
{
var promoAlbums = await _albumService.PromotionalAlbumsAsync();
return View(promoAlbums);
}
...
}
}
Combining Multiple Feature Flags for Razor Views using Tag Helper
If you have any UI elements that you want to put be behind multiple feature flags you can use the <feature>
tag helper to do that. Just like the FeatureGate
attribute, you can pass the requirement type to the tag helper as well. And the allowed values are the same as the filter attribute. It’s All and Any. Look at the example below.
<div class="container">
...
<div class="navbar-collapse collapse d-sm-inline-flex flex-sm-row-reverse">
<ul class="navbar-nav flex-grow-1">
...
<feature name="@Features.Promotions, @Features.PromotionDiscounts" requirement="All">
<li class="nav-item">
<a class="nav-link text-dark" asp-area="" asp-controller="Home" asp-action="Promotions">Promotions</a>
</li>
</feature>
...
</ul>
</div>
</div>
Here we want to hide the Promotions link in the navigation bar when both Promotion
and Promotion.Discounts
flags are not set to false. So, we pass the feature flag names as comma separated strings to the name attribute and set requirement attribute to All.
Summary
In this article we dove a little bit into how you can use multiple feature flags to enable/disable features using different requirement levels. It’s a useful feature to have when you have to make a decision based on multiple feature flags. Let’s look at a few more advanced usages of Microsoft.FeatureManagement library in the next article.
Tags:
You Might Also Like
← Previous Post
Next Post →