Using System Assigned Managed Identity to Access Azure Key Vault from Azure App Service
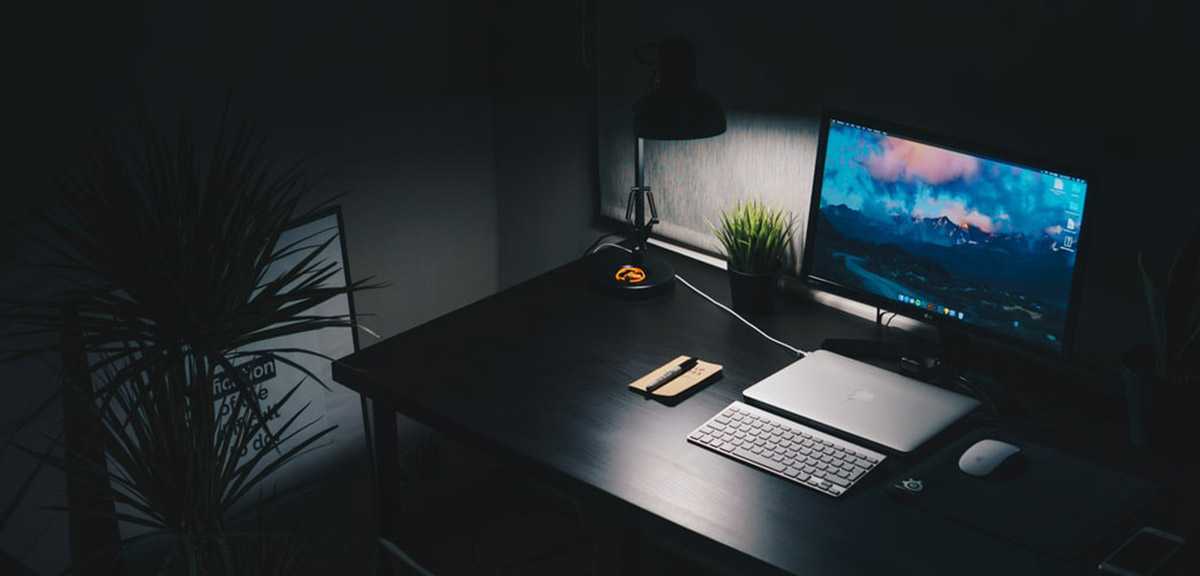
In a previous article, we talk about what Managed Identities for Azure Resource are, the different types of Managed Identities and why we should use Managed Identities. In this article and in few articles to follow, I will show how to use these managed identities, and accessing different services that supports Azure AD Authentication.
In this article, we will look at an ASP.Net Core 2.2 MVC application that will be running on Azure App Service, which needs to access Azure Key Vault to fetch some secrets. And we will use a System Assigned Managed Identity to achieve this.
ASP.Net Core 2.2 MVC Application
The sample application is very basic, it needs to fetch a secret called “TopSecret” from the Azure Key Vault and pass it on to a View Model and show the value on the Home page of the Application. To make this happen we need 2 NuGet packages that needs to be installed in your project.
Microsoft.Azure.KeyVault
– handles the access to the Azure Key Vault and retrieving secrets and keysMicrosoft.Azure.Service.AppAuthentication
– handles the use of Managed Identities in the applications.
Once the NuGet packages are installed, you can use the following code segment to access Key Vault and fetch the secret
// Creating the Key Vault client
var tokenProvider = new AzureServiceTokenProvider();
var vaultClient = new KeyVaultClient(new KeyVaultClient.AuthenticationCallback(tokenProvider.KeyVaultTokenCallback));
We need to provide a Callback function to the KeyVaultClient
to access the Azure Key Vault. The AzureServiceTokenProvider
gives us an implementation of this Callback function that calls the Azure Instance Metadata Service and gets the Access token that needs to be sent to the service you want to access.
The full Controller Action looks something like this.
public async Task<IActionResult> Index()
{
var vaultName = _configuration["AppSettings:KeyVaultName"];
var secretName = _configuration["AppSettings:SecretName"];
var vm = new HomeViewModel();
try
{
// Creating the Key Vault client
var tokenProvider = new AzureServiceTokenProvider();
var vaultClient = new KeyVaultClient(new KeyVaultClient.AuthenticationCallback(tokenProvider.KeyVaultTokenCallback));
// Get the secret
var secret = await vaultClient.GetSecretAsync($"https://{vaultName}.vault.azure.net/secrets/{secretName}");
vm.SecretValue = secret.Value;
}
catch (Exception ex)
{
vm.IsError = true;
vm.ErrorMessage = ex.Message;
}
return View(vm);
}
The changes we need to do the application is now completed. Next we need to publish the application to the App Service instance. Once published, the next steps we will complete using the Azure Portal.
Enabling System Assigned Managed Identity on Azure App Service
Go to your App Service instance and navigate to Settings > Identity and on the Identity blade on the System Assigned tab click on Status toggle and enable it to On. And once you click on Save a system assigned managed identity will be created for you on the Azure AD with the Same name of the App Service Instance.
Now the system assigned identity is enabled on the App Service instance. Next you need to add the Identity that we just enabled as an Access Policy in to Azure Key Vault so that the application can fetch the secrets. To do that, go the Azure Key Vault instance and under the Access Policy section click on Add button. Then you need to select the Service Principal, and search for the App Service name, that will show us the automatically created System Assigned managed identity. Select that identity and give it Secret List and Get permissions and Save.
Once that is done, that is all you need to do to enable a System Assigned managed identity on Azure App Service, and use it to access Azure Key Vault to retrieve secrets. Now if you navigate to the App Service URL, you should be able to see that the Application displays the secret that was retrieved from Azure Key Vault on the home page.
Summery
In this article we discussed how to use Microsoft.Azure.Services.AppAuthentication
NuGet package to use Managed Identities to get access token to access Azure Key Vault, and then we enabled System Assigned managed identity on Azure App Service and used that identity to access Azure Key Vault.
You Might Also Like
← Previous Post
Azure Managed Identities, What? Why? & When?
June 08, 2019
Next Post →