Configuring Dependency Injection for Azure Function V2 Using The New Microsoft.Azure.Functions.Extensions NuGet Package
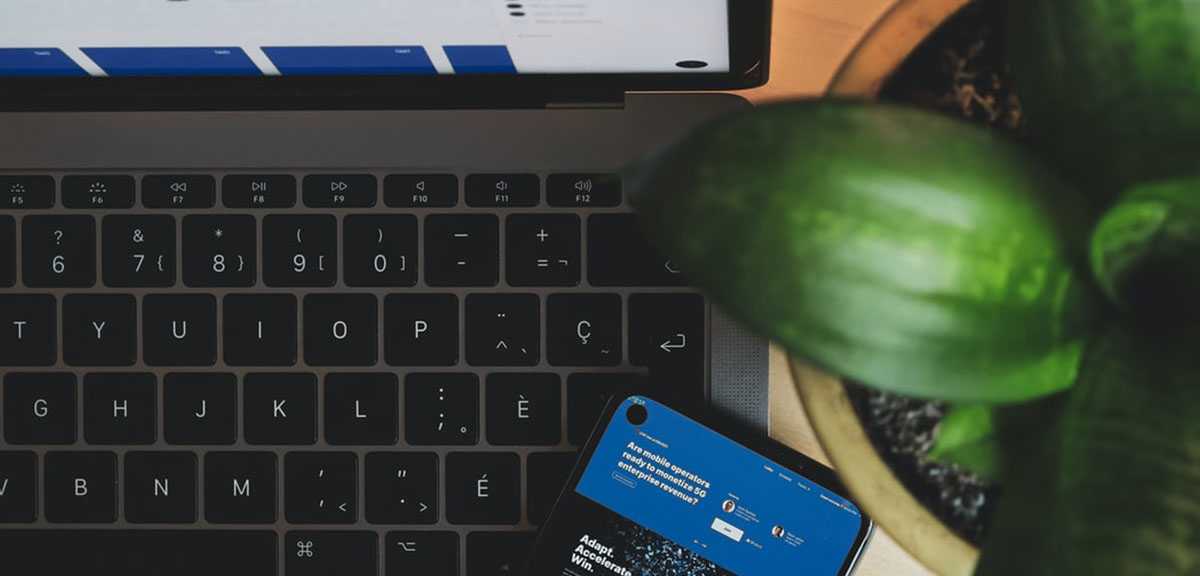
Configuring dependency injection for Azure Functions previously required a bit more work that we would have liked. But now, Microsoft has released the support for using ASP.Net Core Dependency Injection to Azure Functions V2 and the ability to configure it pretty easily. They released the Microsoft.Azure.Functions.Extensions
NuGet package that supports configuring Dependency Injection quire easily using a FunctionStartup
. In this article we’ll see how it can be configured in to a simple Timer Triggered function.
I’ve created a Time Triggered function using Visual Studio 2019. Also, I have added a Service Project which is a .Net Standard 2.0 Class Library project that contains the Services I want to use. In there, I have a small service called HelloService
with the contract IHelloService
. That is, it for the structure of the project, let’s get in to what we need to do to Inject the HelloService
in to my Timer Triggered function.
You need to make sure that the Microsoft.NET.Sdk.Function NuGet package is of the version 1.0.28 or higher. Then after that you need to install the Microsoft.Azure.Functions.Extensions
NuGet package.
To start registering dependencies for the function you need to create a Startup class for the function. This Startup class inherits from a FunctionStartup
class and needs override the abstract method Configure()
. Azure Functions host creates an instance of IFunctionsHostBuilder
and will pass that instance to the Configure()
method. Also, you need to add an assembly attribute to the Startup class to mark the Startup class to be used in the function startup.
In the instance of IFunctionsHostBuilder
instance there is IServiceCollection
property with the name Services where you register your dependencies. So, I want to register my IHelloService
as a dependency and this is how we do it. The full Startup class is given below.
[assembly: FunctionsStartup(typeof(Startup))]
namespace Function.DependencyInjection.Timer
{
public class Startup : FunctionsStartup
{
public override void Configure(IFunctionsHostBuilder builder)
{
builder.Services.AddScoped<IHelloService, HelloService>();
}
}
}
Now the dependency registration is complete. The dependency registration works exactly same like how ASP.Net Core dependency registration works. So, this should be very familiar for you.
Now we can Inject the dependencies in to our function class using Constructor Injection like we are used to. My function code looks something like this.
namespace Function.DependencyInjection.Timer
{
public class TimeFunction
{
private readonly IHelloService _helloService;
public TimeFunction(IHelloService helloService)
{
_helloService = helloService;
}
[FunctionName("TimeFunction")]
public void Run([TimerTrigger("0 */5 * * * *")]TimerInfo myTimer, ILogger log)
{
log.LogInformation($"OUTPUT: {_helloService.SayHello("Kasun Kodagoda")}");
}
}
}
By default, the Function class and the Run method is created as static. This was a big problem since we are not able to use Constructor Injection and we had to resort to Property Injection with Service Locator or Method Injections. But thankfully with this change, you can remove the static modifier from both the class and the method.
With these changes done, you can now run the application and see that when the Timer is triggered the function will use its dependency and call the SayHello()
method and you can see the output in the console window.
Summary
In this article we describe how we can configure dependency injection in Azure Functions V2 using the new Microsoft.Azure.Function.Extension NuGet package and how it works. The source code for this example is available on GitHub using the following link
You Might Also Like
← Previous Post
Next Post →
Premium Hosting Option for Azure Functions
July 06, 2019