Building Apps with Aurelia: #2 Getting Started – Hello Aurelia
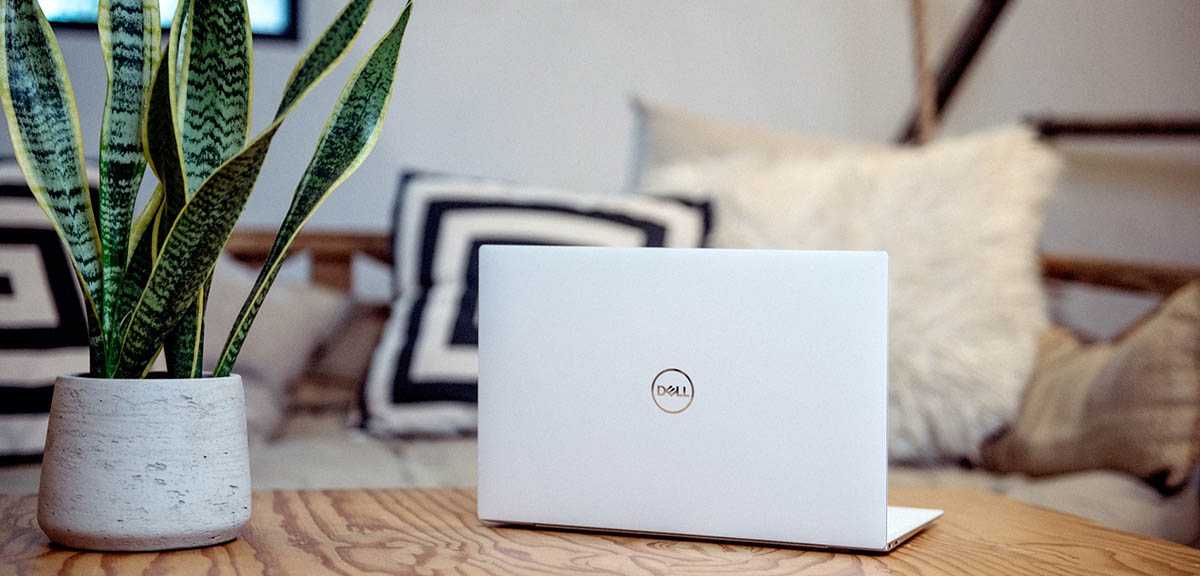
Hey guys, we will diving in to the world of Aurelia, with the simplest possible app we can build. The customary Hello World or in this case Hello Aurelia app.
Without further ado, let’s get in to it shall we? Create a folder on your disk for the project. I’ll name it as Hello Aurelia. Open up a command line and navigate in to the Hello Aurelia folder. We are gonna start off by installing JSPM (JavaScript Package Manager) locally in to this folder. If you have followed the Series Introduction post, you should have JSPM installed globally so this seems a little redundant. But reason to do this is that the machine you are deploying this codebase may or may not have JSPM installed there. So its always good to have JSPM included in the source code itself. :) So install JSPM by typing in the following in to the command line.
npm install jspm --save-dev
This will install JSPM and its dependencies in to the node_modules folder. Next up we need to initialize JSPM to start building the Aurelia app. To do that type in the following command
jspm init
This will start the initialization process by asking you some questions. Look at the screenshot bellow. It will ask to create a Package.json file, ask to enter the base URL for the server, a name for the jspm_package folder yada yada yada.. Accept all the defaults for this step. But in the last question regarding which transpiler to use, make sure bable is the options by default before accepting. Or else type in bable and hit enter. Note: If you are using TypeScript for your development, you should select TypeScript as the transpiler for the last question. Since we are not using TypeScript here, we will accept the defaults (bable in this case). After the final question jspm will initialize and create the config.js file with the settings that we defined.
Next up, we need to use jspm to install Aurelia and Aurelia Bootstrapper in to the project folder. To do that, type in the following command.
jspm install aurelia-framework aurelia-bootstrapper
this will install Aurelia in to the folder and we are ready to build our Hello Aurelia App.. :) Fire up your favorite code editor and create an index.html file in the root of the directory. This will include the base HTML and the references to system.js from JSPM to load the modules and config.js that JSPM init created which sets up the JSPM and bable environments. Also this will also include a line of javascript that kicks off the Aurelia app. Finally the body tag will have an aurelia-app attribute from Aurelia which marks this as an Aurelia app. So the HTML in the index.html file should look like this.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello Aurelia</title>
</head>
<body aurelia-app>
<script src="jspm\_packages/system.js"></script>
<script src="config.js"></script>
<script> System.import('aurelia-bootstrapper'); </script>
</body>
</html>
Next we need to create a place to put our HTML view and JavaScript view models. Let’s create a folder called src in the root directory. And then we need to tell JSPM to look for modules in the src folder. To do that open up config.js file and in the paths section and drop in the following line of code.
"*": "src/*.js",
After this line of code the SystemJs module loader will look for modules in the src folder. And the path section of the config.js file should look something like this
paths: {
"*": "src/*.js",
"github:*": "jspm_packages/github/*",
"npm:*": "jspm_packages/npm/*"
},
Next up we need to create the root view model. By default Aurelia looks for root view models called App. So we need to create this App view model and the associated view. In the later posts we will learn how to override this and use our own view model as the root view model. But for now, lets go with this. So create a app.js and app.html file in the src folder. Put the following code snippet in to the app.js file
export class App {
constructor() {
this.helloMessage = 'Hello Aurelia!';
}
}
So this App class is going to be the root view model. This has a property called helloMessage which contains the message that we are going to bind to an element in the associated view. Now lets add the following HTML to the app.html view.
<template>
<h1>${helloMessage}</h1>
</template>
When you create a view in Aurelia you need to have the HTML in that view inside a element as the root element. And inside that you can have your HTML structure. ${helloMessage} is the binding syntax that Aurelia uses to bind the properties from its view model to its associated view. So this will display the message stored in the helloMessage property in side an Alright folks.. :D now we have our Hello Aurelia app. It’s time to run it and see if all this works. You can do this anyway you want, but I’m gonna use a npm package called http-server to quickly serve the files and test this thing out. So if you install http-server npm package, type this command in to the command line and serve up your files. This will create a simple http server in your Hello Aurelia folder and you can access it on http://localhost:2222. Navigate to this and you should see Hello Aurelia in the page momentarily :D Yay!!. :) If you open up the developer console and refresh the page you can see Aurelia loading up and some debug lines associated with Aurelia loading its resources. To demonstrate 2 way data binding, let’s do some small changes to this app. Let’s add a property called input to the App view model. Add the following line in to the constructor of the App class. Now the App view model should look something like this. and after that we will bind this to an HTML input element and also bind the same property to a paragraph element to see what is the output. So open up the app.html view and add the following inside the tag after the So now the app.html file should look something like this. Here you will see value.bind=”input” in the input element. This is another way to bind to properties in the view models in Aurelia. You can use .bind to bind properties from the view model to attributes in the HTML elements. Then in the element we use the usual binding syntax to bind to the same input property to display what is being typed in to the text input. Let’s try this out. :) Refresh the browser (Hard reload if you don’t see the changes) and now you should see an input box and whatever you type in to it, you should be able to see it down in a paragraph. :) So there you have it folks, a small, simple Aurelia app to get you started in building apps with Aurelia Framework. In the next few articles we would cover the necessary concepts in Aurelia to further improve your skills in building apps using Aurelia Framework. The Source code can be downloaded from here. ← Previous Post June 04, 2016 Next Post → September 18, 2016 element.
http-server -a localhost -p 2222
this.input = '';
export class App {
constructor() {
this.helloMessage = 'Hello Aurelia!';
this.input = '';
}
}
tag.
<input type="text" value.bind="input"> ${input}
<template>
<h1>${helloMessage}</h1>
<input type="text" value.bind="input"> ${input}
</template>
Building Apps with Aurelia: All Articles
Tags:
You Might Also Like
Building Apps with Aurelia: #1 Series Introduction
Building Apps with Aurelia: #3 Customizing Aurelia Application Startup
Comments