Late Binding using dynamic
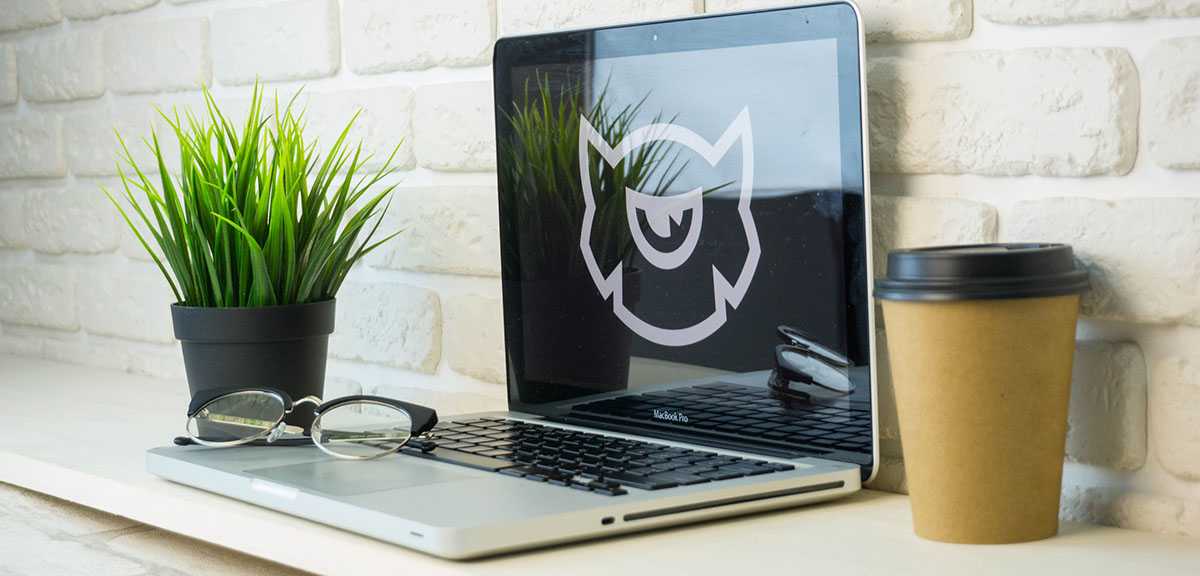
C# is a strictly typed language. In the last post I told you about implicit typing using var. There if you remember I said even if you can let the compiler decide what is the type of a variable you can not dynamically change the type or assign a different type of a value to a variable once defined with either var or normal way. That means it’s static.
But with C# 4.0 (Visual Studio 2010 with .Net 4.0) dynamic type was introduced which let you break that rules. Now using dynamic you can define a variable whose content can be changed at runtime by different types of data. Declarations using dynamic is resolved at runtime. So how do you do that. This is how.. :)
dynamic name = "John";
You also don’t need to initialize the variable at the time of declaration (as it was the case in var). That means you can do this.
dynamic name;
This compiles just fine. Also now you can assign completely different type of value to the variable too. Just like some loosely typed languages like JavaScript, python etc.
dynamic temp = "John Doe";
temp = 100;
temp = new List<string>();
This too compiles without any problems. But this introduces some problems in to your coding. Since C# is strongly typed any errors you do when coding will be detected at compile tome you will be notified. But as soon as you use dynamic the types are resolved at runtime so if you are not careful you will run in to runtime exceptions. So using dynamic gives you flexibility but at a cost, be sure to test out your applications thoroughly before deploying them is you use this dynamic type. So the golden question is?? :D
Why you should use dynamic?
In C# you can work with other loosely types languages like Ruby, Python inside your C# application. Those languages are dynamically typed. So you can use a statically types C# to execute dynamically typed code from those languages. Take a look at IronPython and IronRuby which can be installed through Nuget Package manager.
Also you can use dynamic in situations where you don’t really know what type of objects that may return or you can’t clearly deduce what type you have to work with. I ran in to this problem when i was developing a Windows Phone app where I load few lists from the phones storage (IsolatedStorage to be precise :D ) and I have to select some items using the id. There were different types of lists. Since the functionality was the same apart from the type of list I wanted to refactor my code so I can have one method that do it all.
There was a static method that loads a list from the storage that belongs to a particular type. The method call is like this.
Storage.LoadListFromDisk<T>(listPath); // listPath is the path to the file.
Since the return type may differ my GetItemByID method looks like this. And it uses the dynamic type to accept the return value from the above method.
public static List<object> GetItemByID<T>(string id, string listPath) {
dynamic list = Storage.LoadListFromDisk<T>(listPath);
if (list != null)
{
foreach (var item in list)
{
if (item.ID.Equals(id))
{
// TODO code here.
}
}
}
// TODO Code here
}
Here I need to be sure that the property ID (which I check using item.ID ) needs to be there, coz if not it will result in a runtime exception. Since I know for sure that the ID is there I can safely use it. You may understand the above code or not, it doesn’t really matter :D What matters is that you understand the usage of dynamic and late binding so you can use it if required.
You Might Also Like
← Previous Post
Sharpen Your C#: Implicit Typing
December 14, 2014
Next Post →
Protect Your USB Drive From Autorun Viruses
December 18, 2014